ここでは、書籍「フラクタル: 混沌と秩序のあいだに生まれる美しい図形 アルケミスト双書」のp.22,23に掲載されている、6つのストレンジアトラクターを再現してみました。
Contents
エノンのアトラクター
今回描いたエノンのアトラクターです。
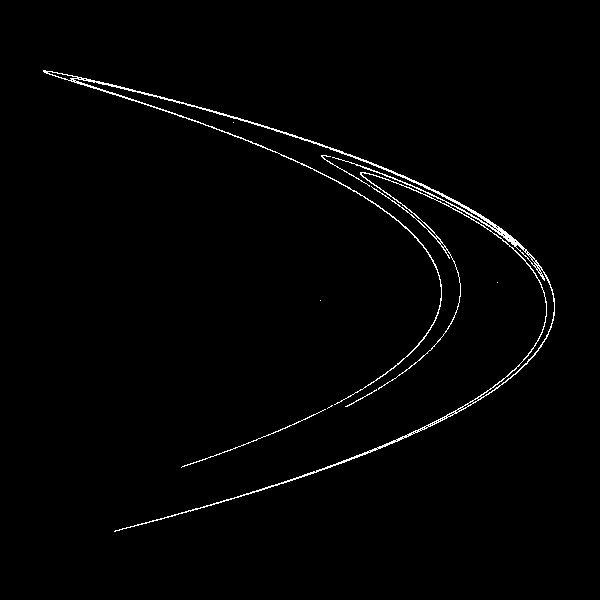
エノンのアトラクターの非線形方程式
エノンのアトラクターを描くための非線形方程式は以下となります。\[ x \to 1+y-1.4x^2, \ \ y \to 0.3 x \]
プログラムコード
今回作成したエノンのアトラクターのプログラムコードを示します。なお、今回は横軸(\(x\)軸)と縦軸(\(y\)軸)の縮尺比を3:1にして描いています。また、最初の座標を\((0.1,0)\)とし、反復回数を200000回にしています。
int iteration_num = 200000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width/2.0,height/2.0);
stroke(255,255,255);
float x_scale = width/3.0;
float y_scale = height/1.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
// 非線形変換
next_x = 1.0+y-1.4*pow(x,2);
next_y = 0.3*x;
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}
ダフィング・アトラクター
次に描いたダフィング・アトラクターです。
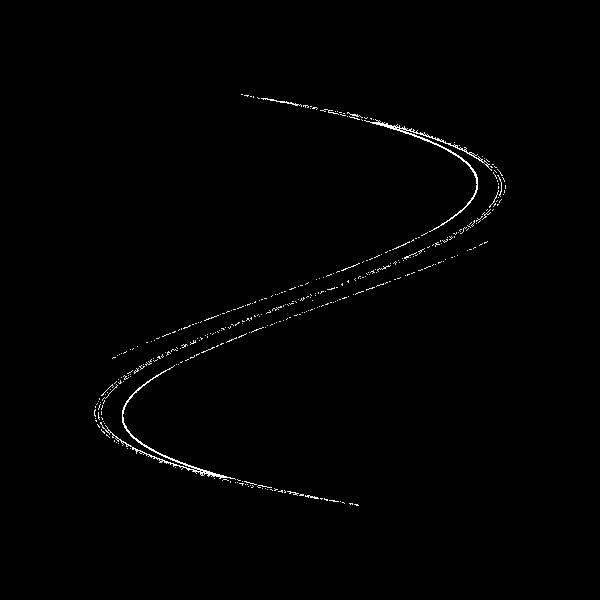
ダフィング・アトラクターの非線形方程式
ダフィング・アトラクターを描くための非線形方程式は以下となります。\[ x \to 2.75x-0.2y-x^3, \ \ y \to x \]
プログラムコード
今回作成したダフィング・アトラクターのプログラムコードを示します。最初の座標を\((0.1,0)\)とし、反復回数を200000回にしています。
int iteration_num = 200000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width/2.0,height/2.0);
stroke(255,255,255);
float x_scale = width/5.0;
float y_scale = height/5.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
next_x = 2.75*x-0.2*y-pow(x,3);
next_y = x;
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}
ティンカーベル
次はティンカーベルです。
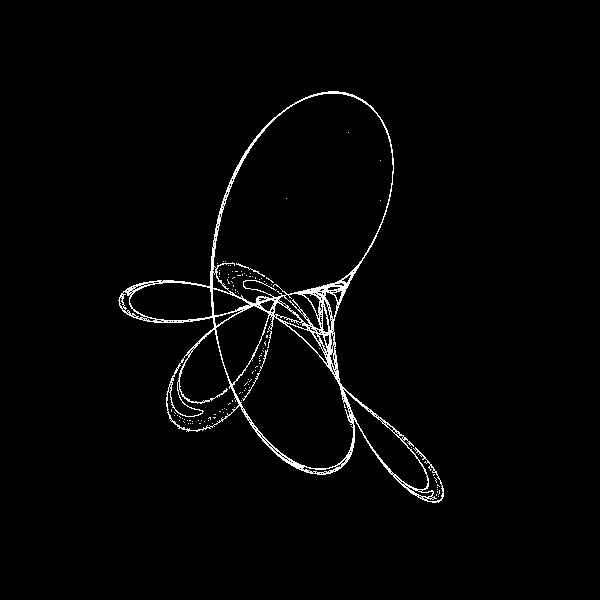
ティンカーベルの非線形方程式
ティンカーベルを描くための非線形方程式は以下となります。\[ x \to (x^2-y^2)+0.9x-0.6y, \ \ y \to 2xy + 2x +0.5y \]
プログラムコード
今回作成したティンカーべルのプログラムコードを示します。最初の座標を\((0.1,0)\)とし、反復回数を200000回にしています。また、図形は全体的に中心からずれているので、座標系の中心位置を多少ずらしています。
int iteration_num = 200000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width*3.0/5.0,height/3.0);
stroke(255,255,255);
float x_scale = width/3.0;
float y_scale = height/3.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
next_x = (pow(x,2)-pow(y,2))+0.9*x-0.6*y;
next_y = 2.0*x*y+2.0*x+0.5*y;
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}
デ・ヨング・アトラクター
次はデ・ヨング・アトラクターです。
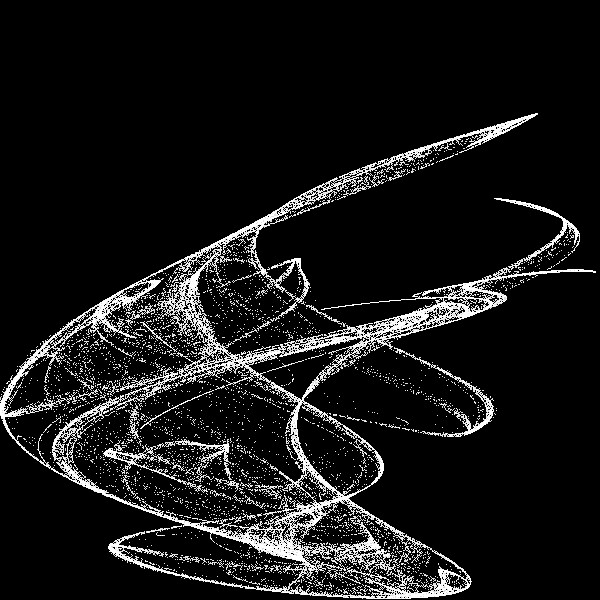
デ・ヨング・アトラクターの非線形方程式
デ・ヨング・アトラクターを描くための非線形方程式は以下となります。\[ x \to \sin (2y) – \cos (2.5 x), \ \ y \to \sin (x) – \cos (y) \]
プログラムコード
今回作成したデ・ヨング・アトラクターのプログラムコードを示します。最初の座標を\((0.1,0)\)とし、反復回数を200000回にしています。
int iteration_num = 200000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width/2.0,height/2.0);
stroke(255,255,255);
float x_scale = width/4.0;
float y_scale = height/4.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
next_x = sin(2.0*y)-cos(2.5*x);
next_y = sin(x)-cos(y);
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}
リントンの幽霊
次はリントンの幽霊です。
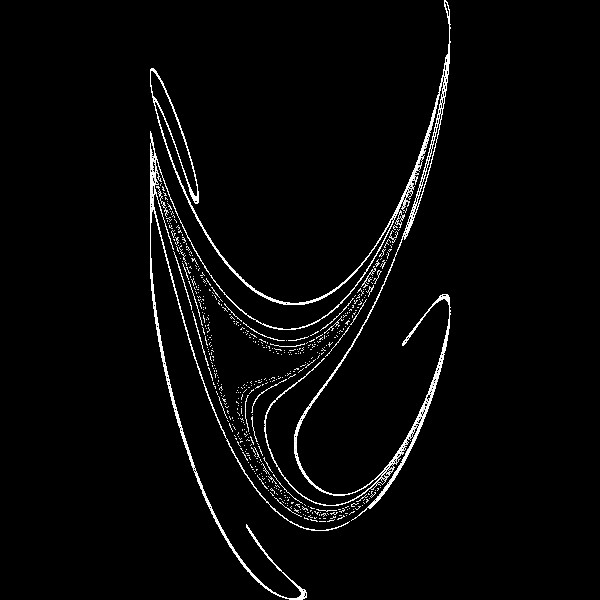
リントンの幽霊の非線形方程式
リントンの幽霊を描くための非線形方程式は以下となります。\[ x \to \sin (1.2y), \ \ y \to -x – \cos (2y) \]
プログラムコード
今回作成したリントンの幽霊のプログラムコードを示します。最初の座標を\((0.1,0)\)とし、反復回数を200000回にしています。
int iteration_num = 200000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width/2.0,height/2.0);
stroke(255,255,255);
float x_scale = width/4.0;
float y_scale = height/4.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
next_x = sin(1.2*y);
next_y = -x-cos(2.0*y);
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}
古典的なバリー・マーティンのアトラクター
最後は、古典的なバリー・マーティンのアトラクターです。
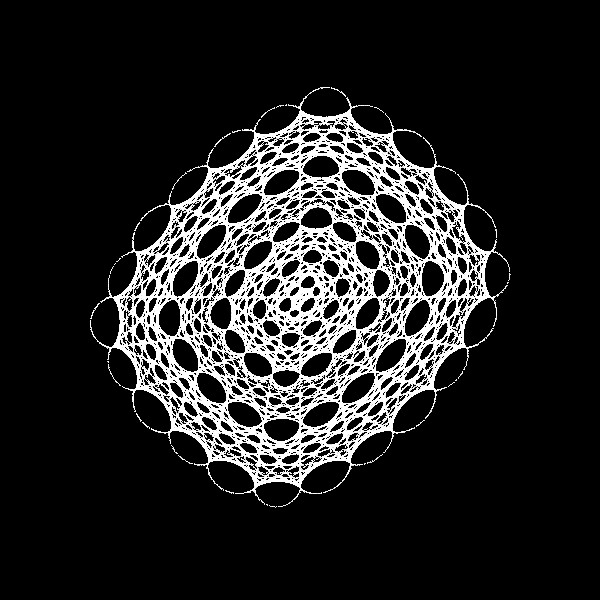
古典的なバリー・マーティンのアトラクターの非線形方程式
古典的なバリー・マーティンのアトラクターを描くための非線形方程式は以下となります。\[ x \to y – \mathrm{sgn}(x) \times \sqrt{|(4x-0.5)|}, \ \ y \to 1-x \]
プログラムコード
今回作成した古典的なバリー・マーティンのアトラクターのプログラムコードを示します。最初の座標を\((0.1,0)\)とし、反復回数を700000回にしています。
int iteration_num = 700000; // 反復回数
void setup(){
size(600,600);
background(0,0,0);
translate(width/2.0,height/2.0);
stroke(255,255,255);
float x_scale = width/200.0;
float y_scale = height/200.0;
float x = 0.1;
float y = 0.0;
float next_x, next_y;
point(x*x_scale, -y*y_scale);
int iter = 0;
while(iter<iteration_num){
next_x = y - sqrt(abs(4.0*x-0.5))*x/abs(x);
next_y = 1.0-x;
x = next_x;
y = next_y;
point(x*x_scale, -y*y_scale);
iter++;
}
}